Understanding Object-Oriented Programming (OOP): Concepts and Examples
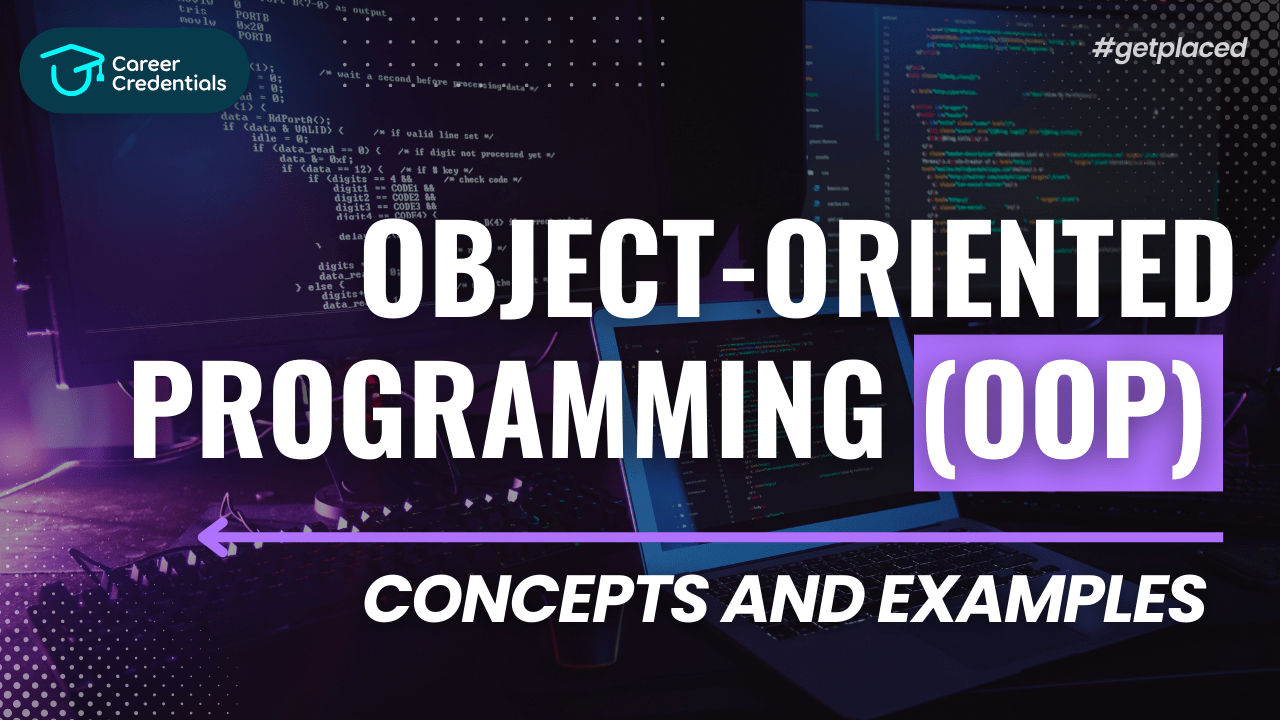
Object-Oriented Programming (OOP) is a paradigm that has revolutionized software development. It revolves around the concept of "objects," which are instances of classes that encapsulate data (attributes) and behavior (methods). This paradigm brings several benefits such as code reusability, modularity, and easier maintenance. Let's delve into some core OOP concepts and provide examples in both C++ and Java.
Core Concepts of OOP
1. Encapsulation
Encapsulation is the bundling of data (attributes) and methods (functions) that operate on the data into a single unit, called a class. This unit hides the internal state of the object from the outside world, and only allows access through defined methods. This helps in data protection and ensures that the object's internal representation is safe and secure.
2. Inheritance
Inheritance is a mechanism where a new class (derived class) is derived from an existing class (base class). The derived class inherits all the properties and behaviors (methods) of the base class. This promotes code reuse and allows for creating a hierarchy of classes.
3. Polymorphism
Polymorphism means "many forms" and allows objects of different classes to be treated as objects of a common parent class. It enables a single interface to represent different underlying forms (classes). There are two types of polymorphism: compile-time (method overloading) and runtime (method overriding).
To know in depth, go through Method Overriding vs Method Overloading By Dr. Amar Panchal
4. Abstraction
Abstraction focuses on hiding the implementation details while showing only the necessary features of an object. Abstract classes and interfaces are used to achieve abstraction, where abstract classes have some concrete methods along with abstract methods, and interfaces only have abstract methods.
For a deeper understanding of all these concepts, watch Pillars Of OOPS Explained By Dr. Amar Panchal
Example in C++
Let's consider an example of a simple banking system using OOP concepts in C++:
#include <iostream>
#include <string>
using namespace std;
// Bank Account Class
class BankAccount {
private:
string ownerName;
double balance;
public:
BankAccount(string name, double initialBalance) {
ownerName = name;
balance = initialBalance;
}
void deposit(double amount) {
balance += amount;
}
void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
cout << "Insufficient funds!" << endl;
}
}
void display() {
cout << "Account Owner: " << ownerName << endl;
cout << "Balance: $" << balance << endl;
}
};
int main() {
// Create Bank Account objects
BankAccount acc1("John Doe", 1000);
BankAccount acc2("Jane Smith", 500);
// Perform operations
acc1.display();
acc1.deposit(500);
acc1.withdraw(200);
acc1.display();
acc2.display();
acc2.withdraw(1000); // Insufficient funds
acc2.display();
return 0;
}
In this C++ example, we have a BankAccount
class with attributes for owner's name and balance. It has methods for depositing, withdrawing, and displaying account details.
Example in Java
Now let's implement the same banking system example in Java:
// BankAccount Class
class BankAccount {
private String ownerName;
private double balance;
public BankAccount(String name, double initialBalance) {
ownerName = name;
balance = initialBalance;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
System.out.println("Insufficient funds!");
}
}
public void display() {
System.out.println("Account Owner: " + ownerName);
System.out.println("Balance: $" + balance);
}
}
public class Main {
public static void main(String[] args) {
// Create Bank Account objects
BankAccount acc1 = new BankAccount("John Doe", 1000);
BankAccount acc2 = new BankAccount("Jane Smith", 500);
// Perform operations
acc1.display();
acc1.deposit(500);
acc1.withdraw(200);
acc1.display();
acc2.display();
acc2.withdraw(1000); // Insufficient funds
acc2.display();
}
}
Here, in Java, we define the BankAccount
class with similar functionalities. We create objects of BankAccount
, perform operations, and display the account details.
These examples illustrate how OOP concepts like encapsulation, inheritance, polymorphism, and abstraction can be applied to real-world scenarios, providing a clearer structure and organization to the code. OOP promotes code reusability, easier maintenance, and enhances the overall design of software systems.
Confused About Your Career?
Don't let another opportunity pass you by. Invest in yourself and your future today! Click the button below to schedule a consultation and take the first step towards achieving your career goals.
Our team is ready to guide you on the best credentialing options for your aspirations. Let's build a brighter future together!
Empower Yourself. Elevate Your Career at Career Credentials Where Education meets Ambition.