Tailwind CSS Explained: A Beginner's Guide
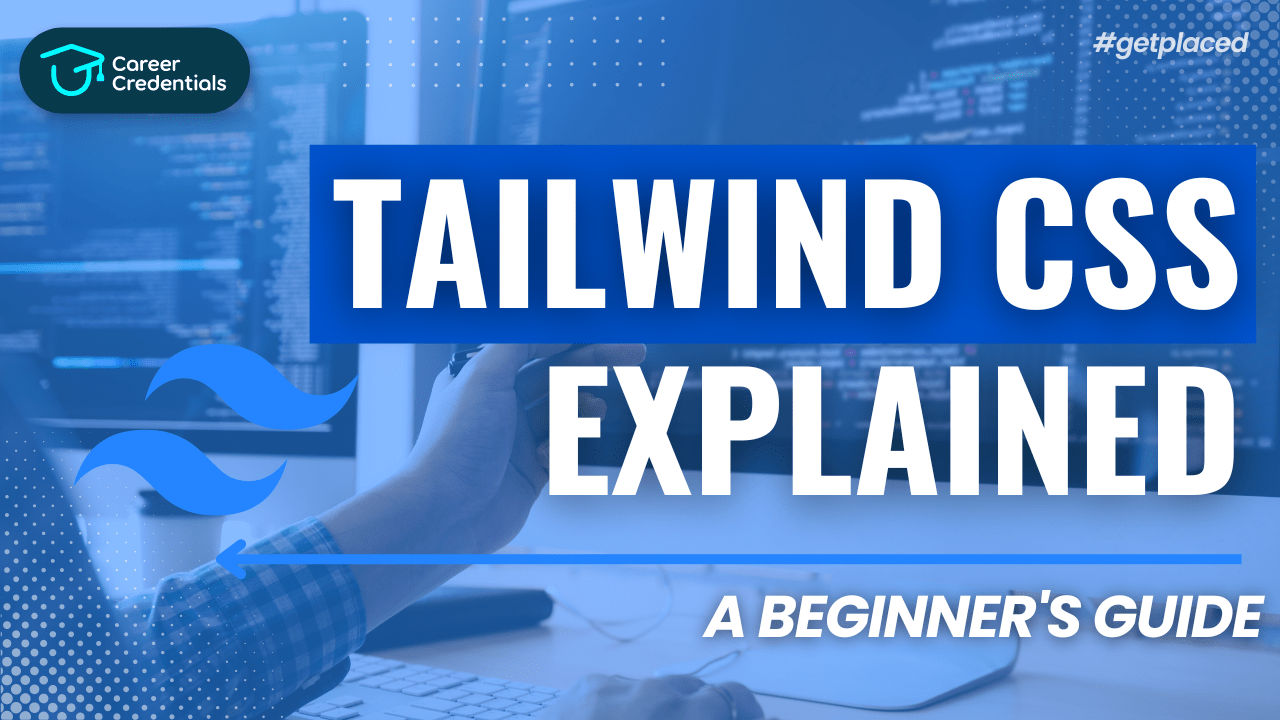
Introduction
Writing CSS can be challenging. It can be frustrating to capture your own ideas or the designs you get from your design team. Many developers have faced this pain at some point in their careers.
But there's good news: it's time to learn about a tool that takes away a lot of the burden from us. And no, it's not Bootstrap – it's called Tailwind CSS.
While Tailwind has been around for a while now, you might not have come across it yet. Maybe you haven't heard about it, or you're unsure whether learning a new CSS tool will really make your life easier. Given the numerous ways to write CSS out there – Vanilla CSS3, LESS, SCSS, Bootstrap, styled-components, Windi CSS, and more – it's understandable to hesitate.
This guide aims to help you understand Tailwind CSS and its benefits, so you can confidently say, "This is it. This is the one."
Enough chit-chat. Let's dive straight in.
What is Atomic CSS?
Before jumping into Tailwind CSS, let's understand what Atomic CSS is. According to CSS Tricks, "Atomic CSS is the approach to CSS architecture that favors small, single-purpose classes with names based on visual function."
In simpler terms, it's about creating classes that achieve a single purpose. For example, let's make a bg-blue
class with the following CSS:
.bg-blue {
background-color: rgb(81, 191, 255);
}
Now, if we add this class to an <h1>
tag, it will get a background color of blue with the specified RGB value. Here's the HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div><h1 class="bg-blue">Hello world!</h1></div>
</body>
</html>
This HTML will result in a blue background for the "Hello world!" text.
Imagine writing such single-purpose CSS rules and keeping them all in a global CSS file. It's a one-time investment that allows you to use these helper classes anywhere. You just need your HTML file to consume that global CSS file. You can also combine these helper classes in a single HTML tag.
An Example of Atomic CSS
Let's create a CSS file with the following rules:
.bg-blue {
background-color: rgb(81, 191, 255);
}
.bg-green {
background-color: rgb(81, 255, 90);
}
.text-underline {
text-decoration: underline;
}
.text-center {
text-align: center;
}
.font-weight-400 {
font-weight: 400;
}
And then consume it in our HTML file as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div><h1 class="bg-blue">Hello world 1</h1></div>
<div><h1 class="text-underline">Hello world 2</h1></div>
<div class="text-center">
<h1 class="bg-green font-weight-400 text-underline">Hello world 3</h1>
</div>
</body>
</html>
This will generate the following results:
- "Hello world 1" with a blue background.
- "Hello world 2" with underlined text.
- "Hello world 3" with a green background, underlined text, centered text, and a font weight of 400.
Points to Note:
- Combining Multiple Helper Classes: Look at how I have combined multiple helper classes in line 14 in the
<h1>
tag:bg-green
,font-weight-400
, andtext-underline
. All these styles are applied to the "Hello world 3" text. - Reusability of Helper Classes: Notice how the
text-underline
helper class is used multiple times in lines 12 and 14.
We can add different styles without leaving the HTML page. While we did have to write those helper or utility classes in the global CSS file initially, think of it as an investment. And who knows how many of these single-purpose helper or utility classes we would need if we followed this Atomic CSS architecture.
That's where Tailwind CSS comes in. The concept of Atomic CSS is not new, but Tailwind CSS takes it to another level.
Tailwind CSS – A Utility-First CSS Framework
Tailwind CSS, according to their website, is a "utility-first CSS framework" that provides several opinionated, single-purpose utility classes that you can use directly inside your markup to design an element.
Some of the utility classes I frequently use are:
flex
: Applies Flexbox to a<div>
items-center
: Applies the CSS propertyalign-items: center;
to a<div>
rounded-full
: Makes an image circular, and so on
It's not possible to list all the utility classes because there are so many. The best part is, we don't have to write these utility classes ourselves and keep them in any global CSS file. We get them directly from Tailwind.
You can find a list of all the utility classes Tailwind offers in their documentation. Also, if you're working in VS Code, you can install an extension called Tailwind CSS IntelliSense, which provides auto-suggestions as you type the utility classes.
How to Set Up Tailwind CSS
There are multiple ways to set up Tailwind CSS in your project, all of which are mentioned in their documentation. Tailwind CSS works smoothly with many frameworks like Next.js, React, Angular, and even plain HTML.
For the following hands-on demo, I will use Tailwind CSS with a Next.js application. To set up a Next.js app with Tailwind CSS, use the following command:
With npx:
npx create-next-app --example with-tailwindcss with-tailwindcss-app
Or with yarn:
yarn create next-app --example with-tailwindcss with-tailwindcss-app
Once the project is set up, you can move on to creating a basic card component.
Hands-on Demo
Let's build a card component in a Next.js project.
Card.js file
import React from "react";
const Card = () => {
return (
<div className="relative w-96 m-3 cursor-pointer border-2 shadow-lg rounded-xl items-center">
{/* Image */}
<div className="flex h-28 bg-blue-700 rounded-xl items-center justify-center">
<h1 className="absolute mx-auto text-center text-2xl text-white">
Image goes here
</h1>
</div>
{/* Description */}
<div className="p-2 border-b-2">
<h6>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis
beatae nulla, atque et sunt ad voluptatum quidem impedit numquam quia?
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis
beatae nulla, atque et sunt ad voluptatum quidem impedit numquam quia?
</h6>
</div>
{/* Tech stack used */}
<div className="flex flex-wrap items-center m-2">
<span className="border border-blue-300 rounded-2xl px-2 my-1 mx-1">
#React
</span>
<span className="border border-blue-300 rounded-2xl px-2 my-1 mx-1">
#Redux
</span>
<span className="border border-blue-300 rounded-2xl px-2 my-1 mx-1">
#Javascript
</span>
</div>
{/* Links */}
<div className="flex flex-wrap items-center rounded-b-xl border-t-2 bg-white">
<button className="border rounded-2xl bg-blue-600 text-white shadow-sm p-1 px-2 m-2">
Go to Project
</button>
<button className="border-2 border-blue-600 rounded-2xl text-blue-600 shadow-sm p-1 px-2 m-2">
Github
</button>
</div>
</div>
);
};
export default Card;
This results in a beautifully styled card rendered in the UI.
Advantages of Tailwind CSS
Just-In-Time (JIT) Mode
Prior to Tailwind v3, it would purge unused styles to keep the production build small, between 5-10 kB. However, in a development environment, the CSS could get quite large. With Tailwind v3 and above, the Just-in-Time compiler compiles only the CSS as needed, resulting in lightning-fast build times in all environments and eliminating the need to purge unused styles.
Opinionated and Flexible
Tailwind CSS is opinionated, providing constraints for styling, which can be beneficial. For example, Tailwind offers only 8 variants for box-shadow, ensuring uniformity and simplifying decision-making. However, if you need a custom style
Confused About Your Career?
Don't let another opportunity pass you by. Invest in yourself and your future today! Click the button below to schedule a consultation and take the first step towards achieving your career goals.
Our team is ready to guide you on the best credentialing options for your aspirations.
Let's build a brighter future together!
Empower Yourself. Elevate Your Career at Career Credentials Where Education meets Ambition.
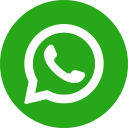
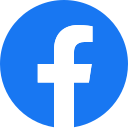
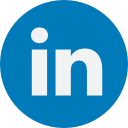