What Is the DOM and Why Is It Crucial for Developers?
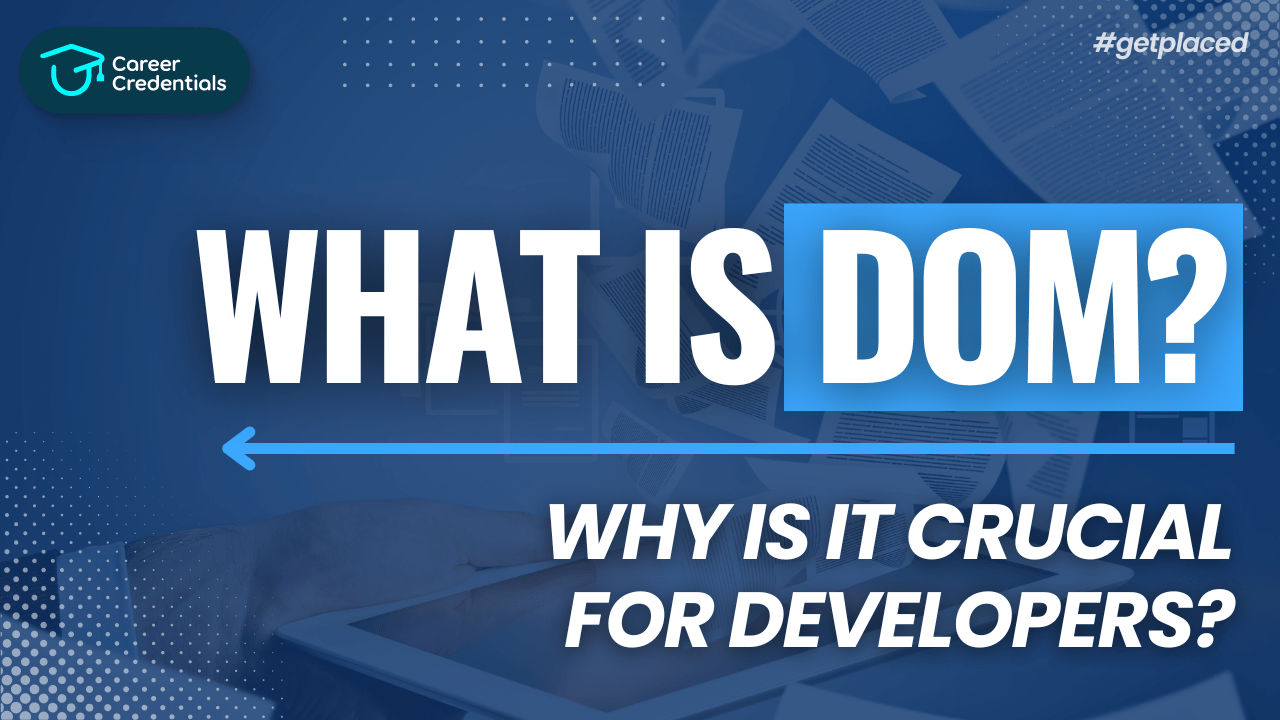
The Document Object Model (DOM) is a fundamental concept in web development, providing a framework for developers to interact with and manipulate the structure of a website. Mastering the DOM is key to creating dynamic and interactive web pages that respond to user actions. In this article, we’ll explore what the DOM is, how it works with JavaScript, and why it's crucial for developers.
What is the DOM?
The DOM, or Document Object Model, is like a blueprint of a website. Just as a blueprint details the layout of a building, the DOM details the structure of a web page. It represents the document as a tree of objects, with each object corresponding to a part of the document.
This structured representation allows developers to navigate and manipulate the website's elements programmatically. For example, they can change the color of a button when a user clicks it or animate images on the page.
Enroll Now: Analysis of Algorithm by Dr. Amar Panchal for a comprehensive understanding of algorithmic analysis and optimization techniques.
The Power of the DOM and JavaScript
JavaScript is a powerful language that enables developers to interact with the DOM. Think of the DOM as a map of the website and JavaScript as a set of tools that can modify the map. Together, they bring websites to life, making them responsive and interactive.
For instance, JavaScript can dynamically change content, style elements, and handle user inputs. By using JavaScript to manipulate the DOM, developers can create engaging web experiences that adapt to user interactions.
Check Out: 53 SQL TCS Interview QnA by Career Credentials for FREE!!
Exploring the DOM Tree Structure
The DOM tree represents a hierarchical structure of a web document. Imagine a website as a book, with the DOM tree serving as the table of contents. Each section in this table of contents corresponds to an element on the web page, organized in a nested manner.
The topmost element, known as the "root," represents the entire document. From the root, branches extend to various elements like headings, paragraphs, images, and more. This tree-like structure makes it easy to locate and manipulate elements on the page.
Check Out: Frontend Roadmap by Career Credentials for FREE!!
Accessing the DOM
To interact with elements on a webpage, you need to access them through the DOM. JavaScript provides several methods to do this, including getElementById
, getElementsByTagName
, querySelector
, and querySelectorAll
. These methods allow you to select elements based on their id, tag name, or CSS selector.
Here’s a practical example:
<div id="student-list">
<div id="student-1" class="student">John</div>
<div id="student-2" class="student">Alice</div>
<div id="student-3" class="student">Bob</div>
</div>
CSS code:
.student {
padding: 40px;
margin-bottom: 10px;
cursor: pointer;
}
.student:hover {
background-color: #f1f1f1;
}
JavaScript code:
let student1 = document.getElementById("student-1");
student1.addEventListener("click", () => {
student1.style.backgroundColor = "lightblue";
});
In this example, JavaScript uses getElementById
to select the element with the id "student-1" and changes its background color to "lightblue" when clicked.
Adding, Removing, and Modifying DOM Elements
Manipulating the DOM isn't limited to just changing styles; you can also add, remove, and modify elements. This capability is essential for creating dynamic content. For example, you might want to add a new button to the page when another button is clicked.
Here’s how you can do it:
HTML code:
<div id="wrapper" class="btn-wrapper">
<button id="create-btn" class="btn">Create new button</button>
</div>
CSS code:
.btn-wrapper {
display: flex;
height: 100vh;
justify-content: center;
align-items: center;
gap: 10px;
flex-wrap: wrap;
}
JavaScript code:
let createButton = document.getElementById("create-btn");
let wrapper = document.getElementById("wrapper");
createButton.addEventListener("click", () => {
let newButton = document.createElement("button");
newButton.innerHTML = "Click me";
wrapper.appendChild(newButton);
});
In this example, a new button element is created and added to the webpage when the "Create new button" button is clicked.
Recap
The Document Object Model (DOM) is a vital tool for web developers, enabling the creation of interactive and dynamic web pages. By understanding the DOM tree and how to access, add, remove, and modify elements, developers can significantly enhance user experiences on their websites.
We’ve explored how the DOM represents a webpage as a tree of objects and how JavaScript methods like getElementById
, getElementsByTagName
, querySelector
, and querySelectorAll
can be used to access specific elements. Additionally, we’ve seen how to add new elements, remove existing ones, and modify element properties to create dynamic web pages.
Conclusion
Mastering the DOM is essential for any web developer aiming to create modern, interactive websites. The DOM provides the foundation for understanding how web pages are structured and manipulated. By leveraging the power of the DOM and JavaScript, you can create engaging, responsive websites that provide a seamless user experience. So, don't hesitate to experiment with the DOM and see what creative solutions you can develop. Happy coding!
Confused About Your Career?
Don't let another opportunity pass you by. Invest in yourself and your future today! Click the button below to schedule a consultation and take the first step towards achieving your career goals.
Our team is ready to guide you on the best credentialing options for your aspirations.
Let's build a brighter future together!
Empower Yourself. Elevate Your Career at Career Credentials Where Education meets Ambition.
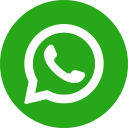
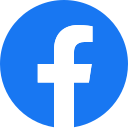
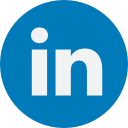