Creating and Managing Threads in Java: Step-by-Step Examples
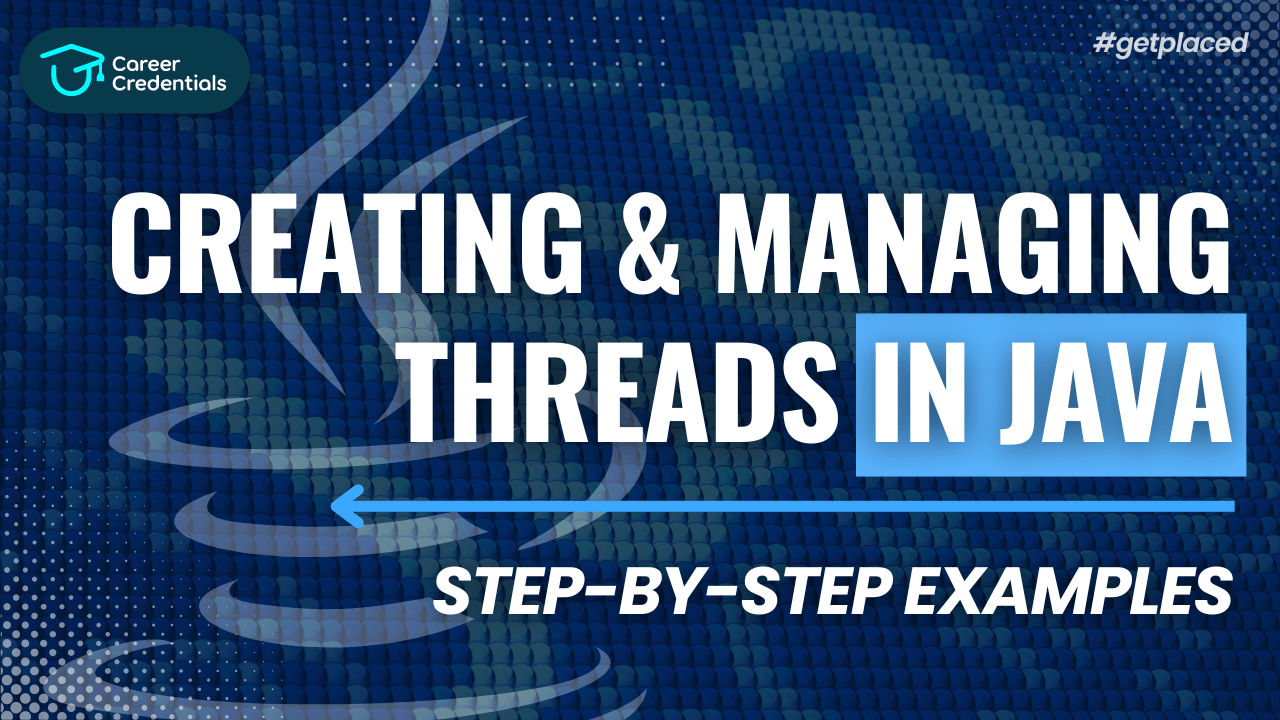
In today's fast-paced digital world, efficiency and performance are paramount. Java, a versatile and powerful programming language, offers robust mechanisms to handle multiple tasks simultaneously. This is where threads come into play. Threads in Java allow you to perform complex operations concurrently without interrupting the main flow of your program. This article delves deep into the creation and management of threads in Java, providing step-by-step examples and a thorough understanding of the concepts involved.
Enroll Now: Core Java by Dr. Amar Panchal to master Java programming fundamentals and advanced concepts.
What are Threads in Java?
Threads are lightweight processes within a program that enable multitasking. They allow multiple operations to occur in parallel, thus speeding up the execution of programs. Each thread runs independently, sharing the same memory space. However, despite this shared memory, an exception in one thread does not impact the functioning of others.
Also Read: Why Learning Java Can Boost Your Career by Career Credentials
Benefits of Using Threads
- Improved Performance: By running multiple operations concurrently, threads can significantly enhance the performance of applications.
- Efficient Resource Utilization: Threads allow better utilization of CPU resources by ensuring that idle time is minimized.
- Responsiveness: In applications like games or interactive software, threads help maintain responsiveness by performing background operations without blocking the main thread.
Multitasking in Java
Multitasking refers to the ability to execute multiple tasks simultaneously. In Java, multitasking can be achieved in two ways:
- Process-Based Multitasking: This involves executing heavy, independent processes. Switching between processes is time-consuming due to their resource-intensive nature.
- Thread-Based Multitasking: This involves executing lightweight threads within a single process. Switching between threads is quicker and more efficient compared to process-based multitasking.
Thread Lifecycle in Java
A thread in Java can exist in several states during its lifecycle. Understanding these states is crucial for effective thread management:
- New: The thread is created but not yet started.
- Runnable: The thread is ready to run and is waiting for CPU time.
- Running: The thread is actively executing.
- Blocked: The thread is waiting for a resource or event to occur.
- Waiting: The thread is waiting indefinitely for another thread to perform a particular action.
- Timed Waiting: The thread is waiting for another thread to perform an action for up to a specified waiting time.
- Terminated: The thread has completed its execution and is no longer alive.
Thread Methods in Java
The Thread
class in Java provides several methods that are essential for thread management:
public void start()
: Starts the thread and invokes therun()
method.public void run()
: Contains the code that constitutes the new thread.public final void setName(String name)
: Sets the name of the thread.public final String getName()
: Retrieves the name of the thread.public final void setPriority(int priority)
: Sets the priority of the thread.public static void sleep(long millis)
: Pauses the thread for the specified duration.public void interrupt()
: Interrupts the thread.public final boolean isAlive()
: Checks if the thread is alive.
Creating Threads in Java
There are two primary ways to create a thread in Java:
- By Extending the
Thread
Class - By Implementing the
Runnable
Interface
Creating a Thread by Extending the Thread
Class
To create a thread by extending the Thread
class, you need to define a class that extends Thread
and override its run()
method.
public class MyThread extends Thread {
public void run() {
System.out.println("Thread is running.");
}
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start();
}
}
Creating a Thread by Implementing the Runnable
Interface
To create a thread by implementing the Runnable
interface, you need to define a class that implements Runnable
and override its run()
method. Then, instantiate a Thread
object, passing the Runnable
object as an argument, and start the thread.
public class MyRunnable implements Runnable {
public void run() {
System.out.println("Thread is running.");
}
public static void main(String[] args) {
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
}
}
Example Programs
Example 1: Using the Thread
Class
public class ExampleThread extends Thread {
public void run() {
System.out.println("Thread is running using Thread class.");
}
public static void main(String[] args) {
ExampleThread thread = new ExampleThread();
thread.start();
}
}
Example 2: Using the Runnable
Interface
public class ExampleRunnable implements Runnable {
public void run() {
System.out.println("Thread is running using Runnable interface.");
}
public static void main(String[] args) {
ExampleRunnable exampleRunnable = new ExampleRunnable();
Thread thread = new Thread(exampleRunnable);
thread.start();
}
}
Advanced Thread Management
Setting Thread Name and Priority
Threads can be given specific names and priorities to manage their execution order and identification.
public class ThreadManagementExample extends Thread {
public ThreadManagementExample(String name) {
super(name);
}
public void run() {
System.out.println("Thread " + getName() + " is running.");
}
public static void main(String[] args) {
ThreadManagementExample thread1 = new ThreadManagementExample("Thread-1");
ThreadManagementExample thread2 = new ThreadManagementExample("Thread-2");
thread1.setPriority(Thread.MIN_PRIORITY);
thread2.setPriority(Thread.MAX_PRIORITY);
thread1.start();
thread2.start();
}
}
Synchronization
To avoid thread interference and memory consistency errors, Java provides synchronization mechanisms. Synchronized methods or blocks ensure that only one thread can access the synchronized code at a time.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class SynchronizationExample {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
thread1.start();
thread2.start();
thread1.join();
thread2.join();
System.out.println("Final count: " + counter.getCount());
}
}
Check Out: 100 Most Asked Java QnA by Career Credentials for FREE!!
Multithreading in Real-World Applications
Example: Word Processor
In a word processor, multiple threads handle different tasks simultaneously. For instance, one thread checks for spelling errors while another saves the document.
class SpellChecker extends Thread {
public void run() {
System.out.println("Checking spelling...");
}
}
class AutoSave extends Thread {
public void run() {
System.out.println("Saving document...");
}
}
public class WordProcessor {
public static void main(String[] args) {
SpellChecker spellChecker = new SpellChecker();
AutoSave autoSave = new AutoSave();
spellChecker.start();
autoSave.start();
}
}
Example: Gaming
In a gaming application, multiple threads manage different aspects of the game such as rendering graphics, handling user input, and processing game logic.
class GameRendering extends Thread {
public void run() {
System.out.println("Rendering game graphics...");
}
}
class UserInput extends Thread {
public void run() {
System.out.println("Processing user input...");
}
}
class GameLogic extends Thread {
public void run() {
System.out.println("Executing game logic...");
}
}
public class Game {
public static void main(String[] args) {
GameRendering gameRendering = new GameRendering();
UserInput userInput = new UserInput();
GameLogic gameLogic = new GameLogic();
gameRendering.start();
userInput.start();
gameLogic.start();
}
}
Check Out: Java Notes by Career Credentials for FREE!!
Conclusion
Threads in Java are powerful tools that allow developers to create efficient and responsive applications. By understanding the lifecycle of threads, utilizing the Thread
class and Runnable
interface, and implementing synchronization mechanisms, you can effectively manage concurrent tasks in your programs. Whether you're developing a word processor, a game, or a server application, leveraging the power of threads will enhance the performance and responsiveness of your software. Happy threading!
Confused About Your Career?
Don't let another opportunity pass you by. Invest in yourself and your future today! Click the button below to schedule a consultation and take the first step towards achieving your career goals.
Our team is ready to guide you on the best credentialing options for your aspirations.
Let's build a brighter future together!
Empower Yourself. Elevate Your Career at Career Credentials Where Education meets Ambition.
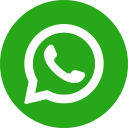
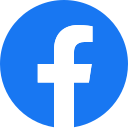
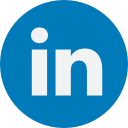