Excelling at LeetCode: Tips and Techniques for Effective Practice

The Struggle is Real
If you've been diligently working through LeetCode problems but feel like you're not improving in interviews, you're not alone. Many aspiring software engineers experience the same frustration. You might be able to come up with solutions, but not the optimal ones. You might run out of time or struggle with difficult questions.
As a career and interview coach, We've seen many of our students face these challenges. The good news is that the issue isn't your intelligence or work ethic—it's your practice strategy.
Common Mistakes in LeetCode Practice
Many people practice LeetCode questions incorrectly because they are unfamiliar with the real-world dynamics of technical interviews. Here's a quick breakdown:
- Interviews are Collaborative: In a real interview, you won't be solving problems alone. You'll be interacting with an interviewer who will observe how you communicate, handle ambiguity, and respond to feedback.
- Questions May Lack Complete Information: Unlike LeetCode, real interview questions might intentionally leave out some details. Interviewers want to see if you'll ask the right questions to clarify ambiguities.
- No Automatic Hints or Test Cases: Interviewers won't always provide hints or test cases. You need to develop the skill to generate and validate your own examples and edge cases.
Check Out: 100 Must-Know Leetcode Problems by Career Credentials for FREE!!
A Better Way: The Six Steps
To improve your LeetCode practice, you need a structured approach. Here are "The Six Steps" that I recommend:
1. Set Up Your Coding Environment
Avoid using a full-featured IDE. Instead, use a whiteboard, pencil and paper, or a simple text editor like Notepad++ or TextPad. This helps simulate the real interview environment where such aids are unavailable.
2. Practice with a Mock Interviewer (Strongly Recommended)
The best practice involves another person acting as your interviewer. Ideally, this should be a fellow software engineer, but even a non-technical person can help. They can provide valuable feedback on your communication and presence. If you must practice alone, hold yourself strictly accountable.
3. Time Your Sessions
Most technical interviews are 45-60 minutes long. Time yourself accordingly. Early in your prep, you might benchmark yourself by working past the time limit to reach an optimal solution, but as you progress, stick to strict time constraints.
4. Articulate the Problem
Have your mock interviewer read the question out loud. Take notes and repeat the question back in your own words to ensure understanding. If practicing alone, read the question once, then hide it and restate it from memory. Focus on key details without rewriting the entire problem statement.
5. Ask Questions and Confirm Assumptions
Always ask questions to clarify the problem, even if you think you understand it. Here are some common questions:
- What is the data type and range of the input?
- Can I expect invalid data?
- How will the input be provided?
- Is the input sorted?
Restate your assumptions clearly. If practicing alone, document your questions and assumptions, then check the LeetCode constraints for answers.
6. Create Example Inputs and Outputs
Work through provided examples and create your own. Treat them like test cases. This helps you understand problem constraints and devise a solution. If practicing with a mock interviewer, they should correct any example that violates constraints.
7. Brainstorm Solutions and Estimate Big-O Complexity
Generate multiple solutions, starting with a brute force approach. Estimate their time and space complexity upfront. Aim for an optimal solution and validate your approach with examples. Discuss potential solutions with your interviewer if possible.
8. Implement the Solution
Implementation should be straightforward if you've planned well. Use clear, verbose variable names and explain each step before writing code. Avoid pseudocode; write actual code. Practicing coding from memory can help strengthen your understanding and coding skills.
9. Test Your Code
Walk through your code line by line to catch bugs or errors. Use your examples to validate the code mentally. Common issues to watch for include:
- Undeclared variables
- Off-by-one errors
- Reversed conditionals
- Null pointer exceptions
10. Optimize
If you haven't reached an optimal solution, brainstorm and implement improvements. Keep refining until the timer runs out.
Enroll Now: Javascript Crash Course by Career Credentials to master JavaScript!
Wrapping Up Your Mock Interview
After your mock interview, document your performance and feedback in a journal. If practicing with a partner, compare your self-assessment with their feedback. Focus on non-technical feedback as well, like communication skills.
Finally, copy your code into the LeetCode editor or an IDE to ensure it compiles and passes tests. Note any failures or missed edge cases for future reference.
Enroll Now: 53 SQL TCS Interview QnA by Career Credentials for FREE!!
Conclusion
By following these steps, you can transform your LeetCode practice into a more effective preparation for real technical interviews. This structured approach not only improves your problem-solving skills but also enhances your ability to communicate and collaborate under pressure. Keep practicing, stay disciplined, and track your progress to excel in your interviews.
Confused About Your Career?
Don't let another opportunity pass you by. Invest in yourself and your future today! Click the button below to schedule a consultation and take the first step towards achieving your career goals.
Our team is ready to guide you on the best credentialing options for your aspirations.
Let's build a brighter future together!
Empower Yourself. Elevate Your Career at Career Credentials Where Education meets Ambition.
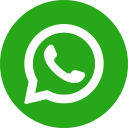
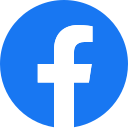
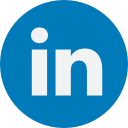